Quickstart Guide
The IMIconnect Conversation system comprises the set of components which makes it easy for developers to integrate a fully featured chat experience within the application.
The details below outlines the additional steps necessary to integrate the Conversation System of the IMIconnect SDK for iOS within a host application and assumes the use of the Xcode.
Prerequisites
Before proceeding further, you are required to integrate the IMIconnect Core SDK for iOS within their application. If not done, please follow the Core Quickstart Guide set-up the MIIconnect Core SDK for iOS.
Note: The Conversation System requires the Core SDK to be correctly configured before the system will function.
Project Setup
Add the UI SDK to your project
- Copy paste the
IMIconnectUISDK.framework
in your project folder. - Open your Xcode project and perform the following steps:
Link IMIconnectUISDK.framework to your project
- Click on your pbxproj in Xcode and go to the General tab.
- In the Embedded Binaries section, click the + sign.
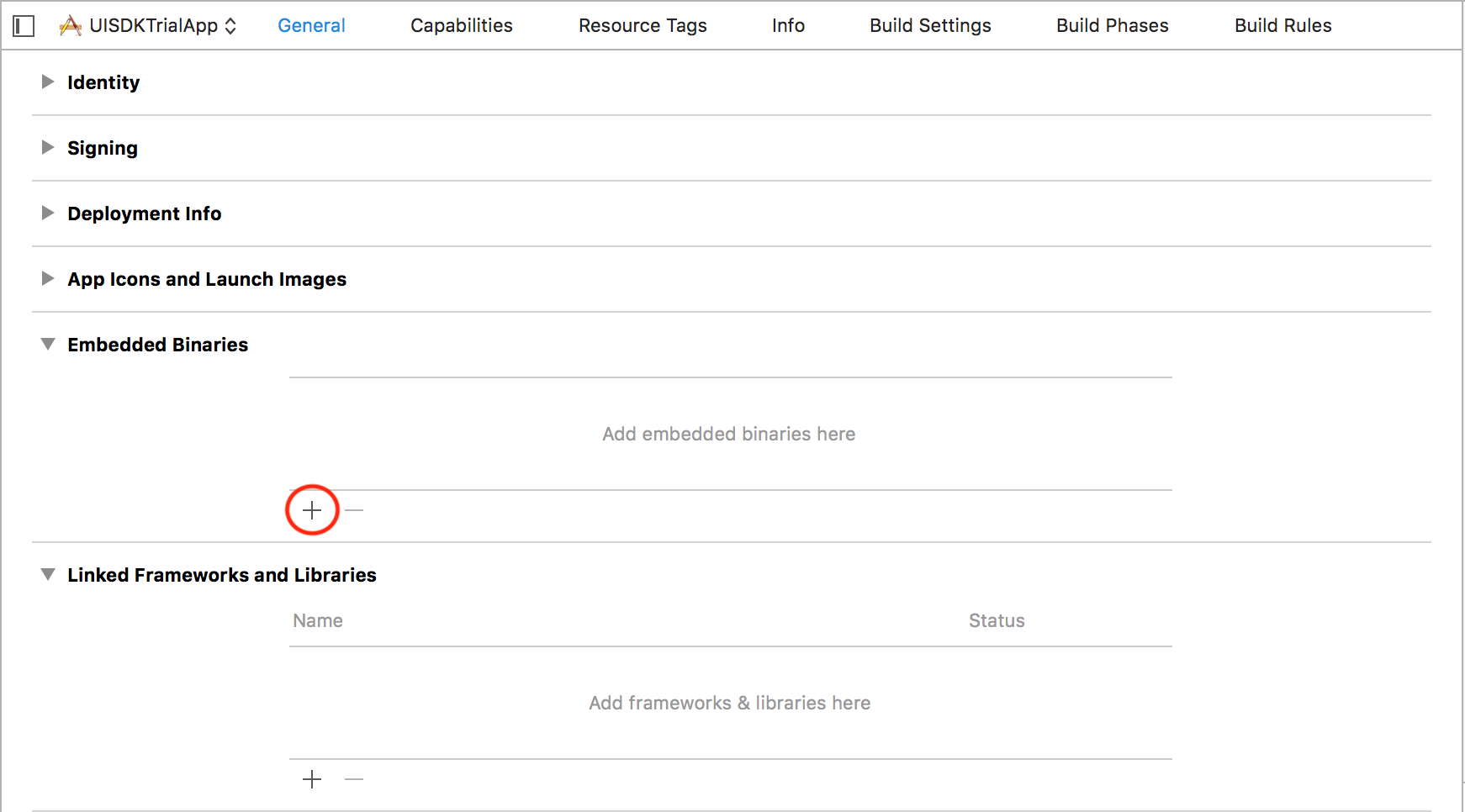
- In Choose items to add window, select Add Other.
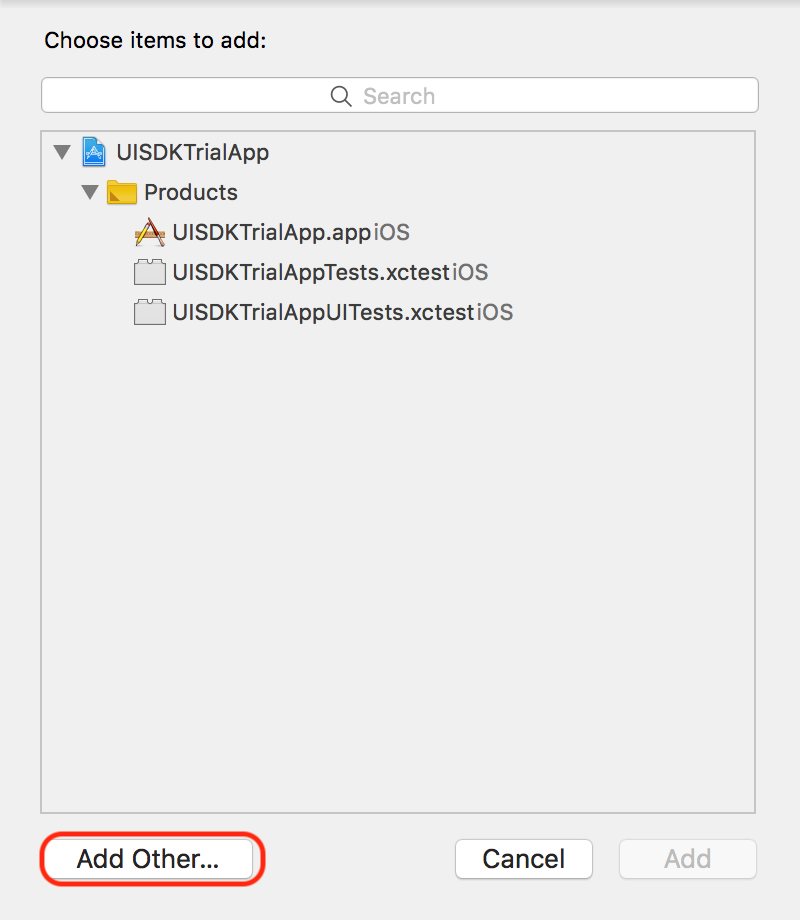
- Select IMIconnectUISDK.framework in your project folder and click Open.
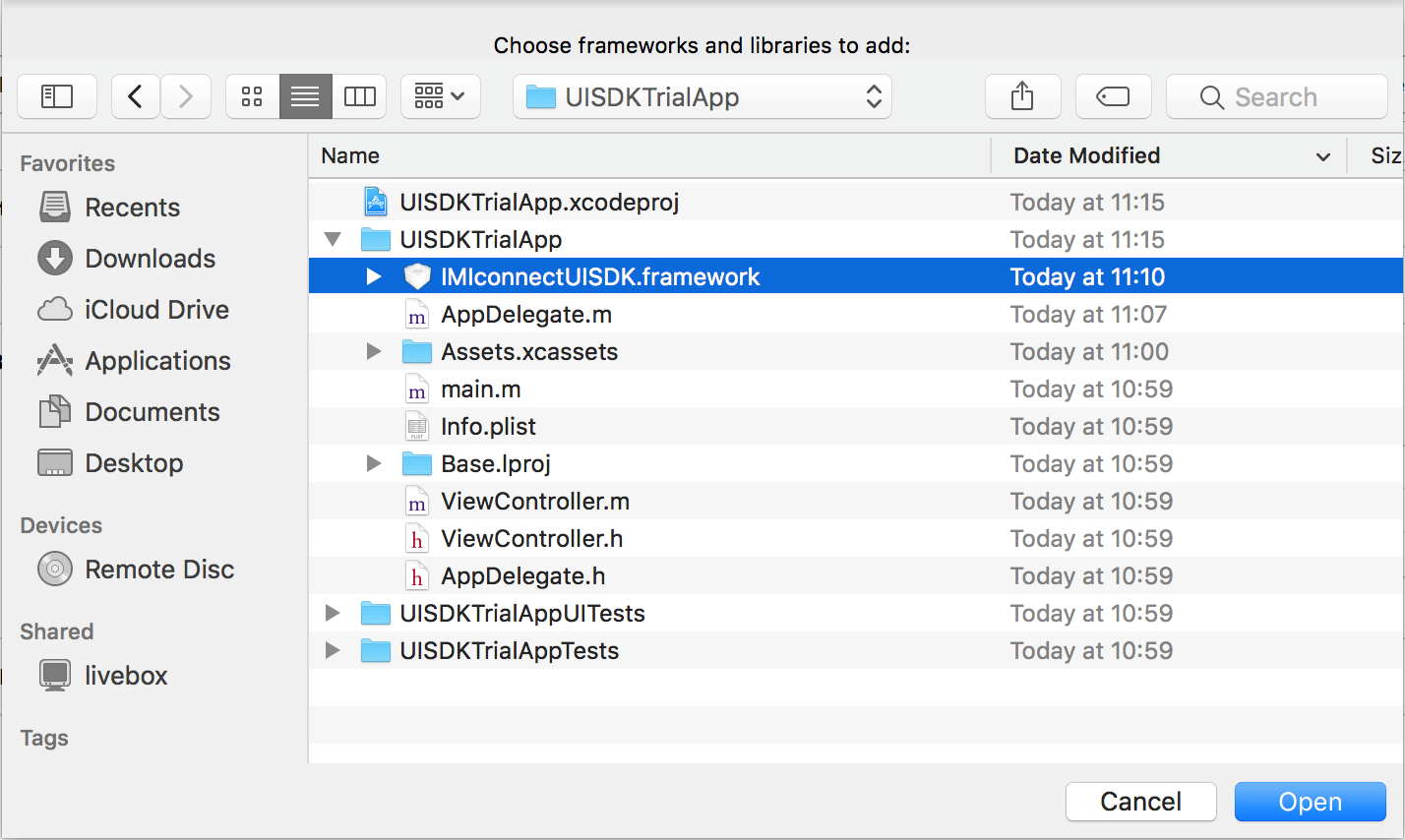
- You should now be able to see
IMIconnectUISDK.framwork
added to the Build phases and in your project structure in Xcode
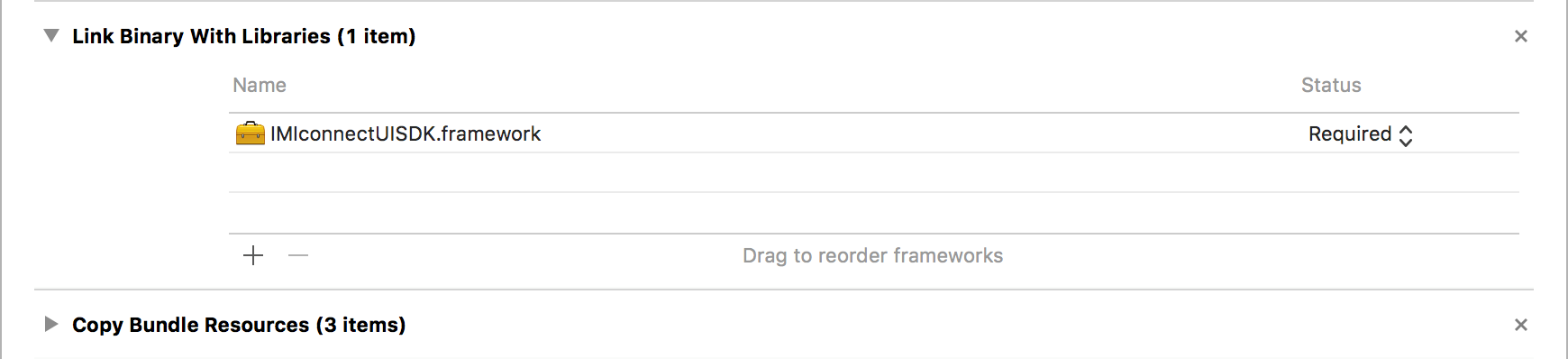
Implementation
Configure a message store
The Conversation System UI components require that a valid message store has been configured prior to the components being used. The Core SDK provides default implementations which may be used out of the box.
The following code creates an ICDefaultMessageStore instance and passes it to ICMessaging to enable message store functionality. You should place this code within your AppDelegate didFinishLaunchingWithOptions: method immediately after the IMIconnect.startup method call.
`[ICMessaging shared].messageStore = [[ICDefaultMessageStore alloc] initWithPassword:@"Your Password"];
Configure Categories
Categories define the contact points within your organisation and allow you to segregate conversations within the platform. Categories are presented to the user as a list and selection of a category is required to start a new conversation. For example, imagine you wish to allow the user to contact a number of different departments, each of these departments would be represented by a category.
You configure categories by creating instances of the ICConversationCategory class like so:
NSMutableArray *categories = [NSMutableArray new]; [categories addObject:[[ICConversationCategory alloc] initWithTitle:@"Sales" streamName:@"sales"]]; [categories addObject:[[ICConversationCategory alloc] initWithTitle:@"Technical support" streamName:@"techsupport"]];
A valid list of categories is required in order to launch the ICConversationViewController within the next section.
Launch the SDK features using storyboard reference
The UI SDK provides several components which can be used to integrate a chat experience within your application, the components you choose to use will depend on how much customisation you require. For the purposes of this guide, we will use the storyboard reference to IMIconnectUISDK.storyboard to see how to easily integrate the components.
Xcode 9 or below:
In your Main storyboard, in the right bar, select the first tab Show the file inspector then at the bottom, select the third tab Show the Object library and select Storyboard Reference.
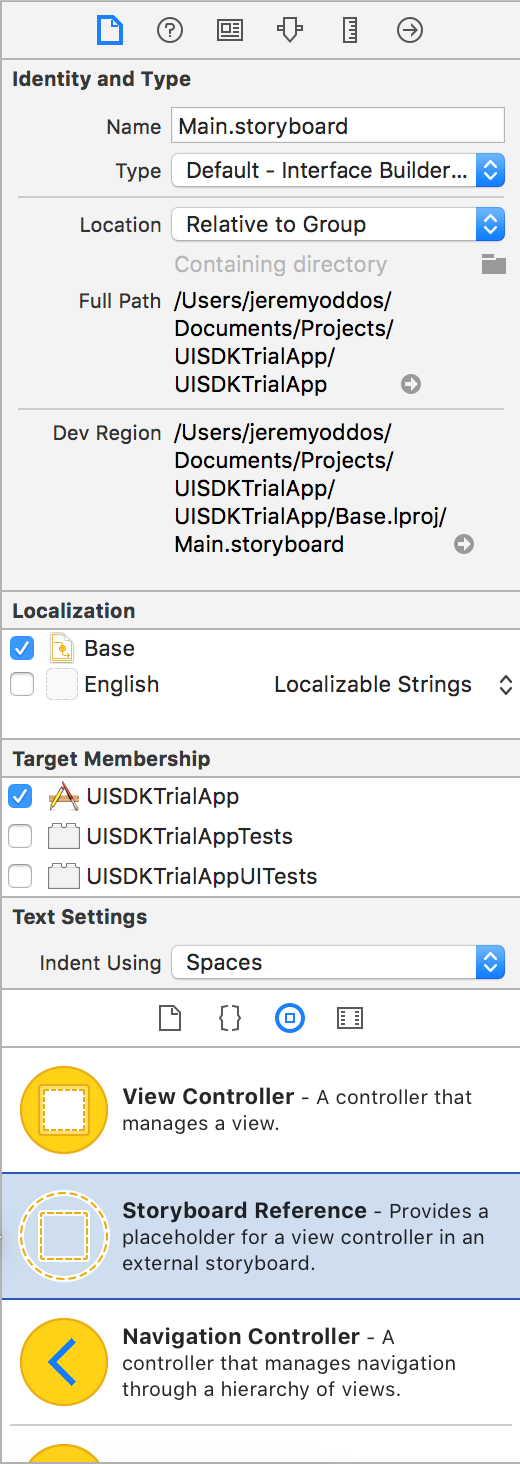
Xcode 10 or later:
If you are using Xcode 10 or later then you can find this Library just beside the Standard Editor on Editing Panel. Click on Library and select Storyboard Reference.
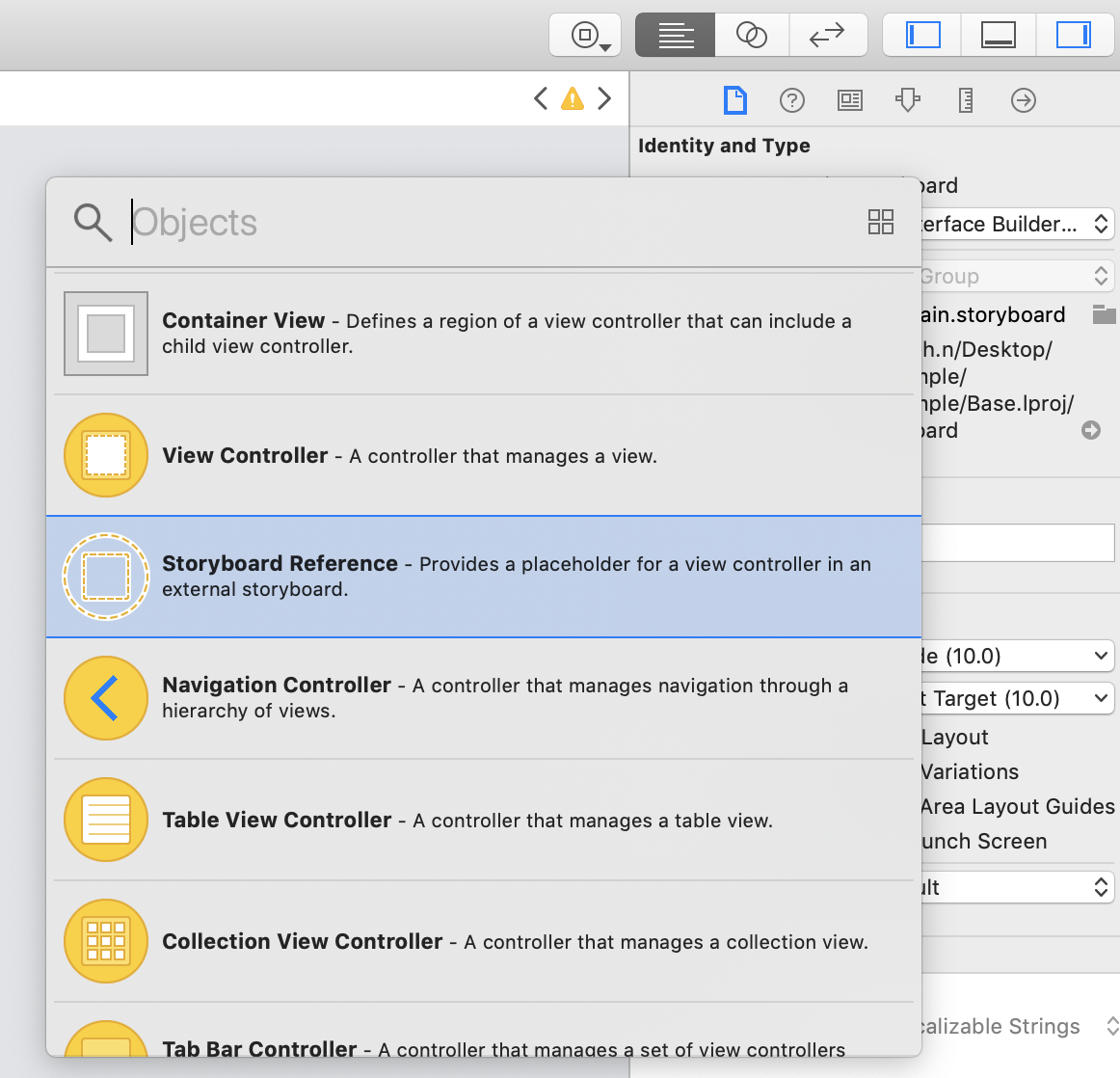
- Drag and drop the storyboard reference in your Main storyboard.
- Select the Storyboard Reference and go to the fourth tab Show the attribute inspector.
- In the Storyboard text field, enter
IMIconnectUISDK
- In the Referenced ID text field, enter
ICConversationsSplitViewController
- In the Bundle text field, enter
com.imimobile.imiconnect.IMIconnectUISDK
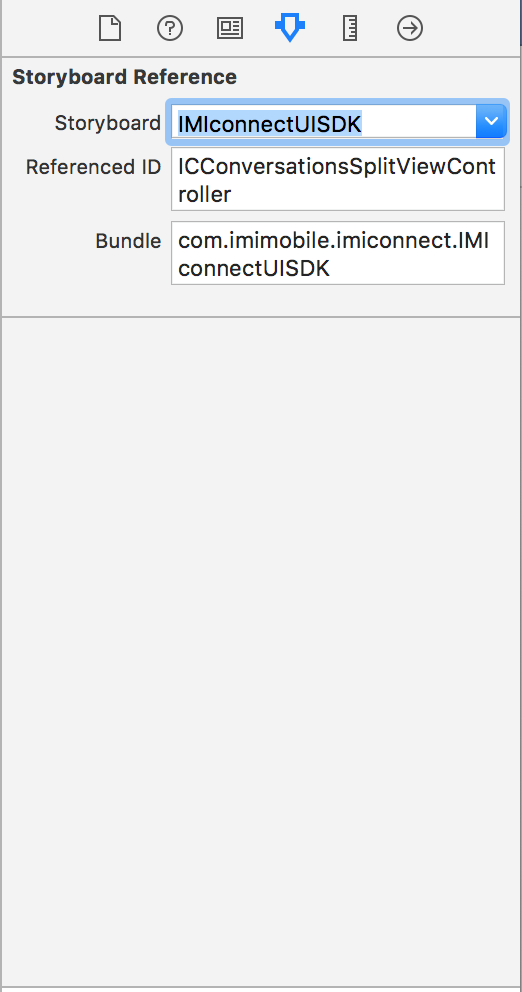
Open a Conversation directly
To launch the ConversationViewController to directly display a conversation use the following method variant:
UIStoryboard *uiSDKStoryboard = [UIStoryboard storyboardWithName:@"IMIconnectUISDK"
bundle:[NSBundle bundleWithIdentifier:@"com.imimobile.imiconnect.IMIconnectUISDK"]];
ICConversationViewController *conversationViewController = [uiSDKStoryboard instantiateViewControllerWithIdentifier:@"ICConversationViewController"];
conversationViewController.thread = <The thread>;```
Note: The thread is exposed by the Core SDK.
##Message synchronization (optional)
To provide the best possible user experience the Core SDK provides synchronization of messages across devices. This is useful to obtain message history when a user installs your application to a new device.
Message synchronization is managed by the Core SDK but will only function when a message store has been set and a valid synchronization policy has been provided.
###Configure synchronization policy
The ICMessageSynchronizationPolicy class allows you to configure various options which limit how much data is synchronized, for the purpose of this guide we will enable full synchronization.
The following code snippet enables synchronization of the full user message history:
```javascript
ICMessageSynchronizationPolicy *policy = [ICMessageSynchronizationPolicy new];
policy.mode = ICMessageSynchronizationModeFull;
[ICMessageSynchronizer setPolicy:policy];```
Updated over 2 years ago