Cordova Android Setup
This Quickstart guide explains the necessary steps required to integrate the Cordova plugin into your Android app. It also provides the steps to set-up an app asset corresponding to your app on IMIconnect platform.
Prerequisites
Component | Requirement |
---|---|
Android Operating System | Android OS 4.0 (API Level 15) and above. |
Software |
|
Accounts |
|
Configuration Tasks
To setup cordova, follow these tasks in sequence:
- Integrate Firebase
- Configure a IMIconnect Mobile Application
- Cordova Installation and Setup
- Code Integration
Integrate Firebase
The IMIconnect SDK leverages Firebase Cloud Messaging for it's push capability. Please follow the official Firebase setup guide, which will take you through the process of configuring a Firebase project, adding gradle dependencies and google-services.json to your Android Studio project.
Important! ( need revision)
If you use the Firebase Assistant, only follow steps 1 & 2, the SDK takes care of the rest, no additional code is required.
Firebase version support
IMIconnect Core plugin 2.0.0 supports Firebase versions 11.0.0 and higher.
Capture the Firebase Server Key
The Firebase Cloud Messaging server key allows IMIconnect to submit push notifications to your application. The following steps will provide directions for obtaining the key, please make a note of the key to use it later for configuration in this guide.
- Open the Firebase Console
- Click the Firebase project to open it. (You created this in the previous section)
- Click the Settings icon, followed by Project Settings.
- Make a note of the Server Key (This is needed within the next section)
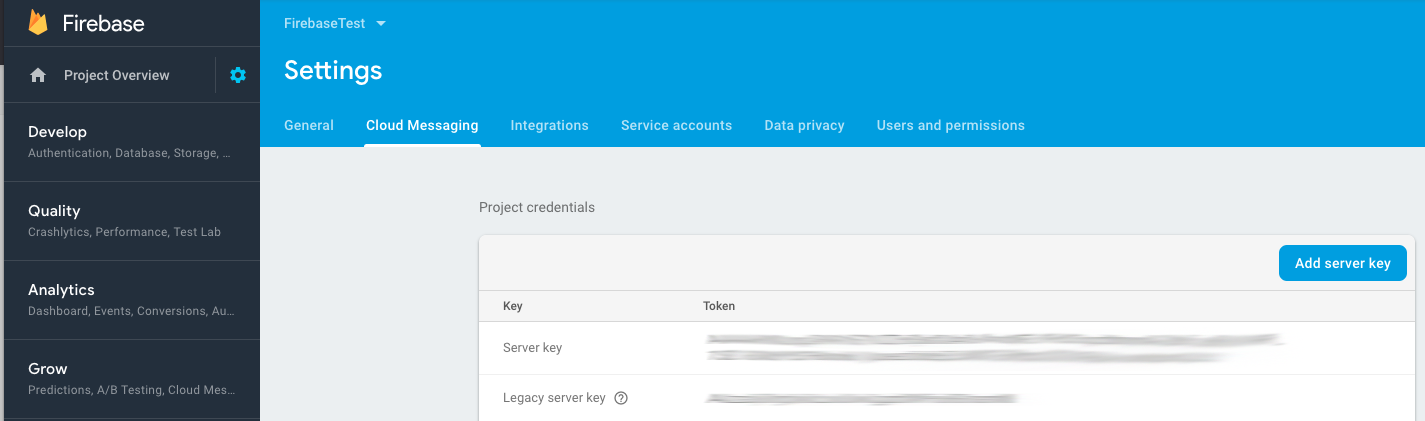
Configure a IMIconnect Mobile Application
In this section you will configure a mobile application within the IMIconnect Portal.
Your account administrator should have been provided a url to the IMIconnect Portal, this url is unique to your account. If you do not have your account url, please contact your account administrator.
- Login to your IMIconnect Portal account using your unique account url.
- From the menu, click Apps.
- Click CONFIGURE APPS > MOBILE / WEB.
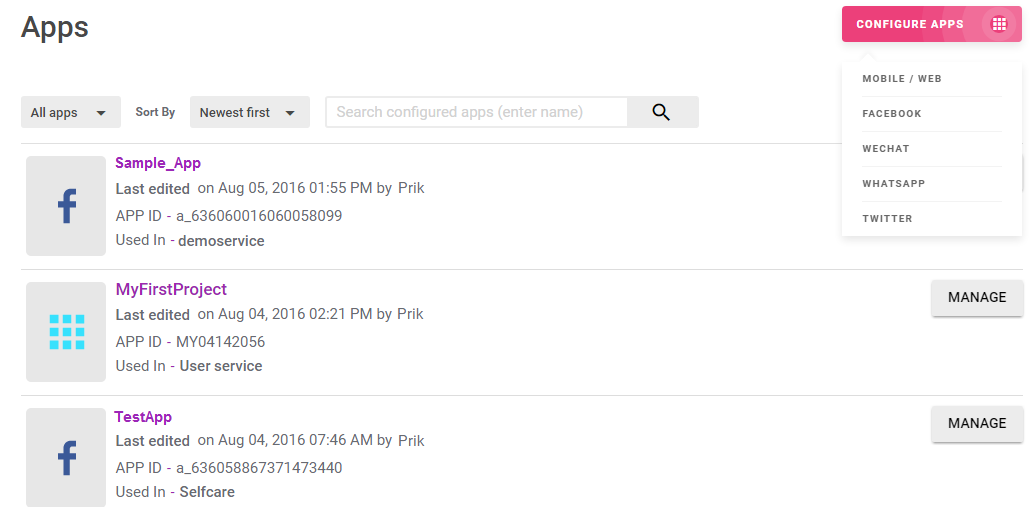
- Enter a name for your application and click NEXT.
- Select 'Mobile' and click NEXT.
- Select the Android platform and click NEXT.
- The Android platform specific features are displayed. Enable the features that you wish to use and configure the settings as appropriate:
Feature | Settings |
---|---|
Real-time messaging | Configure the following:
Note: The RTM Transport and Security settings are common for all the configured platforms of the app. If you set for one platform, the same is applied to all other platforms. Transport protocols Security settings Enable RTM payload encryption to encrypt the RTM payload in transit. |
Push notifications | Enter your FCM Server key within the Push notifications settings box. |
Two factor authentication | Select the Sender ID through which an OTP (One Time Password) is sent. |
Device attributes | To monitor different attributes such as Time Zone, IMEI, RAM, Location and so on. |
- Click SAVE.
- Make a note of the Client Key. This will be used while integrating the IMIconnect SDK within your application.
- Click SAVE.
An overview screen will appear with the newly created application profile and the corresponding App ID.
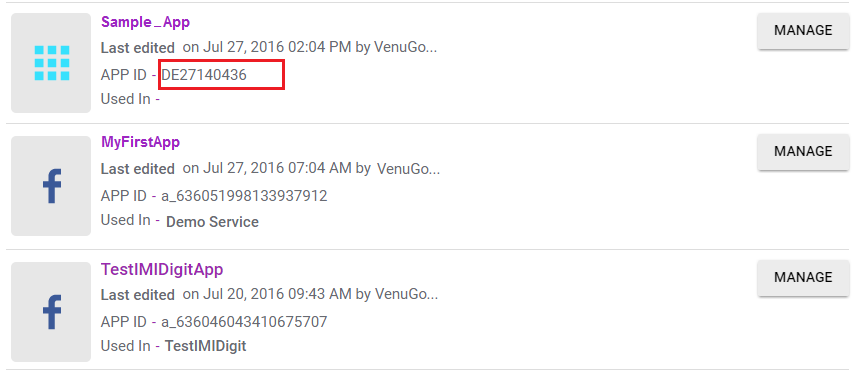
- Make a note of the App ID. This will be used while integrating the IMIconnect SDK within your application.
Cordova Installation
- To setup the Cordova plugin in your machine, you are required to install npm and Node in your machine.
- Once you have npm installed, install the Cordova in your machine using the following command.
$ npm install -g cordova
- Create a Cordova application by executing the command.
$ cordova create myapp com.mycompany.myapp MyApp
In the command above, myApp is the directory name, MyApp is the application name and com.mycompany.myapp is the package name. For more details refer to this link.
Android Installation for Cordova
After you have created the Application successfully, add the Android platform to the application.
- Navigate to the application folder and execute the below command.
$ cordova platform add android
This will create a folder with the name android inside the application path myApp/platforms. - Create the .apk file to test the application using the following command
$ cordova build android
Executing the above command will create .apk file with the name android.debug.apk inside the application path myApp/platforms/android/build/outputs/apk - The application is ready to execute. To run the application, start a new AVD(Android Virtual Device) with the following command
$ android avd
- On executing the command, a pop-up screen is displayed to create a new virtual device.
- Execute the below command to start the emulator and run the .apk file in the emulator
$ cordova emulate android
- Now add the custom plugin to the application.
Navigate to the cordova application folder(i.e myapp) and execute the below command.
$ cordova plugin add <local plugin dir>
Example :
$ cordova plugin add IMIconnectPlugin - To list all the plugins installed in your machine use the following command
$ cordova plugin list
- To remove a particular plugin from the application follow the below command.
$ cordova plugin remove plugin_name
Android Local Setup
- Open android.manifest file and add the below tags inside the manifest tag.
<permission android:name="{ Name of your package}.permission.C2D_MESSAGE" android:protectionLevel="signature" /> <uses-permission android:name="{ Name of your package}.permission.C2D_MESSAGE" />
Example:
<permission android:name="io.cordova.hellocordova.permission.C2D_MESSAGE" android:protectionLevel="signature"/>
<uses-permission android:name="io.cordova.hellocordova.permission.C2D_MESSAGE"/>
- Open build.gradle file and add the below tags inside the buildScript -> dependencies
- Get the google-services.json file from firebase and copy the same in platforms -> androidlocation.
- Configure the appId and clientKey in string.xml which is available in android/res/values/string.xml, as shown below
<string name="app_id">YOUR APPID</string>
<string name="client_key">YOUR CLIENT KEY</string>
5.Now that the application is ready, create the .apk file to test the application by executing the following command.
$ cordova build android
Code Integration
Follow the below steps to integrate IMIconnect Android SDK in your application:
a. Register a Device
b. Receive messages
c. Connect to IMIconnect
d. Listen for connection status events
e. Fetch Topics
f. Subscribe to a Topic
g. Unsubscribe from a Topic
h. Create a Thread
i. Publish a Message
j. Disconnect from IMIconnect
a. Register a device
The user's device must be registered with the IMIconnect platform before other features can be used. To register a device with the platform, create a ICDeviceProfile
instance and invoke the IMIconnect.register
method.
A device profile must always have a unique device id and user id, if you do not supply a user id then the platform will assign one for you. Typically you will want to supply your own user id value that corresponds to a user within your backend systems.
var deviceProfile = new ICDeviceProfile();
deviceProfile.setAppUserId("9999");
deviceProfile.setDeviceId("PROVIDE UNIQUE DEVICE ID");
IMIconnectPlugin.register(
function(result) {
console.log(result);
},
function(error) {
console.log(error);
},
deviceProfile
);
ICDeviceProfile provides a convenience device id implementation that is based on Secure.ANDROID_ID, but you are free to supply your own value instead.
If your solution uses provisioned customer profiles (a.k.a master profiles), you will need to call IMIconnect.updateProfileData to link your device profile to the customer profile. You may do this after successful device registration.
See here for information on provisioning customer profiles.
b. Receive messages
Incoming Push and In-App messages are received by registering a MessageListener
implementation with IMIconnectPlugin.
Implement your c listener as per the below code samples:
IMIconnectPlugin.messageListener(
function(messages) {
var text = "";
for (var i = 0; i < messages.length; i++) {
text += messages[i].getMessage() + "<br>";
}
console.log(text);
});
The following sections are only relevant if you wish to use In-App Messaging.
c. Connect to IMIconnect
To use In-App Messaging you must establish a connection with the IMIconnect platform. Invoke the connect
method to establish a connection and allow messages to be received.
Once the RT feature is enabled in the app asset created on IMIconnect and user registration is done, the App Developer can establish a connection between the app and IMIconnect platform by calling the connect method appropriately. This enables the messages sent from IMIconnect to be received on the app. When the application is running in the background, SDK is disconnected from IMIconnect. While in the disconnected state, incoming In-App messages are not received, however when the application comes to foreground again, SDK will establish a connection with IMIconnect platform and allow messages to be received.
This method will throw an error when In-App Messaging is not enabled for the app or a device is not registered with the SDK.
IMIconnectPlugin.connect(
function(result) {
console.log(result);
},
function(error) {
console.log(error);
}
);
d. Listen for connection status events
Events are raised by the SDK whenever the connection status has changed. To receive these events in your application; implement and register a connectionStatusChangedListener
.
IMIconnectPlugin.connectionStatusChangedListener(
function(result) {
console.log(result);
},
function(error) {
console.log(error);
});
e. Fetch Topics
IMIconnect supports Topic based messaging, use the fetchTopics
method to retrieve a list of topics configured for your application. You can subscribe users, or publish messages to those topics.
Note:
- Topics can be configured by an Admin in admin console of IMIconnect.
IMIconnectPlugin.fetchTopics(function(topics) {
var text = "";
for (var i = 0; i < topics.length; i++) {
text += topics[i].getName() + "<br>";
}
window.alert(text)
}, function(error) {
console.log(error);
}, {
"offset": 0
});
f. Subscribe to a Topic
Invoke the subscribe
method to subscribe the current user to a topic.
IMIconnectPlugin.subscribeTopic(
function (result) {
console.log(result);
},
function (error) {
console.log(error);
},
topicId);
g. Unsubscribe from a Topic
Invoke the unsubscribeTopic
method to unsubscribe the current user from a topic.
IMIconnectPlugin.unsubscribeTopic(
function (result) {
console.log(result);
},
function (error) {
console.log(error);
},
topicId);
h. Create a Thread
All In-App Messages within IMIconnect are grouped by threads. In order to publish messages, you must first create an ICThread
object.
When responding to an incoming message, you can obtain the ICThread
directly from the incoming message.
var thread = new ICThread();
thread.setTitle("MyTitle");
thread.setCategory("MyCategory");
IMIconnectPlugin.createThread(function(thread) {
window.alert(thread.getTitle());
}, function(error) {
console.log(error);
}, thread);
i. Publish a Message
Invoke publishMessage
to publish a message to IMIconnect.
Note:
An ICThread object is obtained by calling the
createThread
method or from a received incoming message.
var message = new ICMessage();
message.setMessage("Test Message");
message.setThread(thread);
IMIconnectPlugin.publishMessage(function(icMessage) {
console.log(JSON.stringify(icMessage.toJSON()));
}, function(error) {
console.log(error);
}, message);
j. Disconnect from IMIconnect
This method is used to disconnect from IMIconnect. Once disconnected, incoming In-App messages will not be received.
This method throws an exception when In-App Messaging is not enabled for the app.
IMIconnectPlugin.disconnect(
function(result) {
console.log(result);
},
function(error) {
console.log(error);
}
);
End
Integration of the IMIconnect plugin is now complete.
Updated almost 2 years ago