Cordova iOS Setup
The quickstart guide contains a detailed step by step process of integrating the Cordova plugin in your iOS app. It also provides steps to configure an app asset corresponding to your app on IMIconnect platform.
Prerequisites
Component | Requirements |
---|---|
OS | iOS 8 or higher. |
Software |
|
Accounts |
|
Configuration Tasks
To use IMIconnect SDK for your Mobile Application,
Cordova Installation
- To setup the Cordova plugin in your machine, you are required to install npm and Node in your machine.
- Once you have npm installed, install the Cordova in your machine using the following command.
$ npm install -g cordova
- Create a Cordova application by executing the command.
$ cordova create myapp com.mycompany.myapp MyApp
In the command above, myApp is the directory name, MyApp is the application name and com.mycompany.myapp is the package name. For more details refer to this link.
Installing the Requirements for iOS
Xcode
-
To continue with the application setup, install Xcode in your machine. If Xcode is not installed you can find the Xcode in two different ways.
- From the App Store, available by searching for "Xcode" in the App Store application.
- From Apple Developer Downloads, which requires registration as an Apple Developer.
-
Once Xcode is installed, several command-line tools need to be enabled for Cordova to run. From the command line, run:
$ xcode-select --install
Deployment Tools
The ios-deploy tools allow you to launch iOS apps from an iOS device. On the command-line, execute the following command to install ios-deploy.
$ npm install -g ios-deploy
iOS Installation for Cordova
Add pinned version of the iOS platform and save the downloaded version to config.xml & package.json
$ cordova platform add ios
Download Cordova SDK
- Login to the IMIconnect platform with the URL provided to you.
- On the left menu, click TOOLS and select Downloads.
The SDK Download page is displayed as shown below.
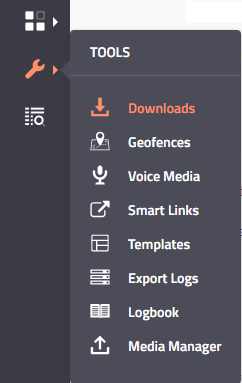
Tools - Download SDK
- Under DOWNLOAD CORE SDK tile, click the Cordova icon as shown below to download the Cordova SDK
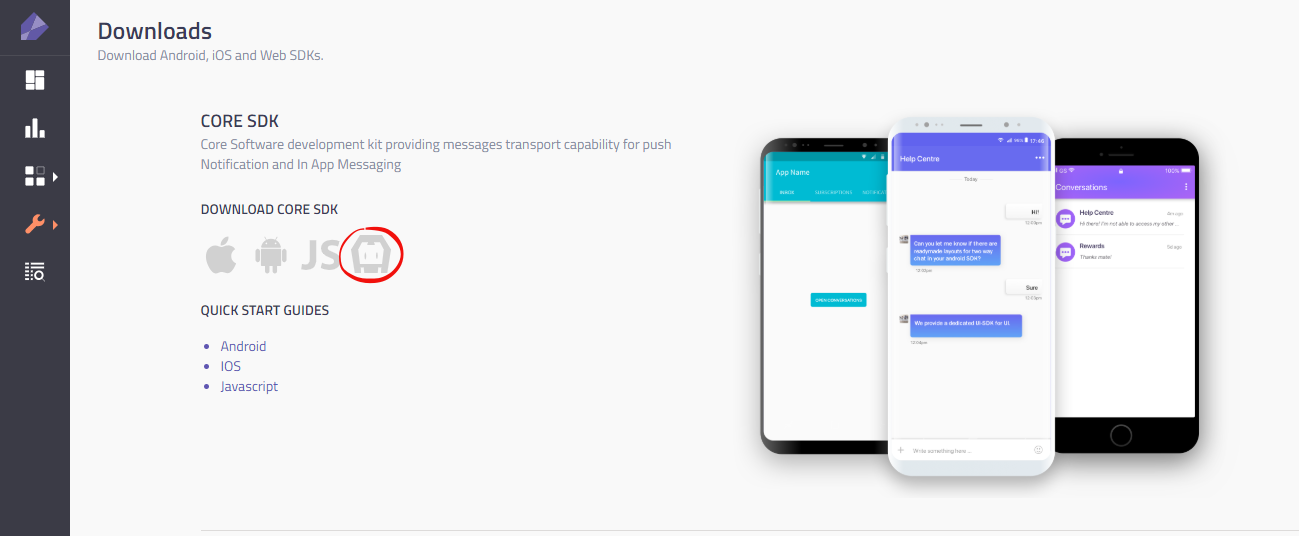
Cordova SDK Download
Adding plug-in
- To add custom plug-in to the application; navigate to the cordova application folder(myApp) and executive the following command.
in the example above, IMIconnectPlugin is the plug-in** name.$ cordova plugin add IMIconnectPlugin
- Use the following command to build the Cordova application.
$ cordova build ios
### Project Setup
To deploy your Cordova application in the iOS simulator
1. Open the workspace file ``(../platforms/ios/MyApp.xcworkspace)`` in Xcode. or you can use the below command line to open the workspace file:
```
$ open ./platforms/ios/MyApp.xcworkspace/
```
2. After opening the workspace file, Make sure the **MyApp** project is selected in the left panel.
Note: Refer [Setup APNs](#section-setup-apns) to create **p12** file using the same identifier.
* After Creating the P12 file. In Application, switch ``on`` the PushNotifications from **Capabilities tab** on the main window.
* Switch ``ON`` the Background Modes, and ``checkmark`` the 'Remote notifications' in the capabilities tab.
3. On the main window, select **General tab** and provide the **Bundle Identifier**(you can change this as per your requirement) and **Signing Details**.
4. Now give the following Framework status as optional in **Linked Frameworks and Libraries** section in the same General tab.
* FileProvider.Framework
* IOSurface.framework
* UserNotificationUI.framework
* UserNotifications.framework
* IMIconnectNotificationServiceExtension.framework
5. Remove IMIconnectNotificationServiceExtension.framework from **Linked Frameworks and Libraries** , and add it on **Embedded Binaries**
6. In order to receive the notifications, enable the Bluetooth permission.
Go to **info** tab and click the **+** icon anywhere on the displayed list. Enter the **key** as **Privacy - Bluetooth Peripheral Usage Description** and **value** as **$(PRODUCT_NAME) Bluetooth Peripheral use**.
7. In order to receive Rich Push Notifications, add NotificationService Extension.
a.Target on pressing "(+)" icon which is available on target section,
b. Refer [Add Notification service](#section-rich-notification-support) for adding extension Target.
8. When adding Extension, you will notice a **New Folder** in your project hierarchy containing three new files ** NotificationService.h ** NotificationService.m** and ** Info.plist**.
* Add **IMIconnectNotificationServiceExtension.framework** manually in the new Folder, the file is available at ``plugin/src/ios/lib``
* In **Info.Plist** of ``your new notificationextension Target`` , add **App Transport Security Setting** Dictionary with **Allow Arbitrary Loads**; Bool value **YES** by selecting **+**.
9. On the **General Tab**,->**Linked Frameworks and Libraries**, set "IMIconnectNotificationServiceExtension.framework" status as **optional**.
10. Now create a Group Identifier, refer [create-an-app-group](#section-create-an-app-group) section in **Quickstart Guide** for more information
11. Switch **ON** the ``AppGroups``, then **checkmark** ``your group identifier`` in the capabilities tab. from main project target, Extension Target as well.
12. Finally, connect the mobile (device) to your machine to see the connected device on the Scheme menu.
13. Click **Run** button from the same toolbar to the left of the Scheme to build, deploy, and executive the application on the connected device.
Now you can see your application is running on the device.
14. In your application level plist add AppId, ClinetKey & groupIdentifier as shown in below image. (Same image which is showing in document as of now)
[block:image]
{
"images": [
{
"image": [
"https://files.readme.io/ed8cf41-img11.jpg",
"img11.jpg",
553,
107,
"#ebebe9"
],
"border": true,
"caption": "Add New Key"
}
]
}
[/block]
Use below code to startup SDK in your main view controller.
[block:code]
{
"codes": [
{
"code": "- (void)viewDidLoad\n{\n [super viewDidLoad];\n // Do any additional setup after loading the view from its nib.\n //Password to generate encryption key for secure preferences\n IMIconnectPlugin *pushHandler = [self getCommandInstance:@\"IMIconnectPlugin\"];\n pushHandler.password = @\"setYourPassword\";\n //SDK initializing\n NSDictionary *infoPlistDict = [[NSBundle mainBundle] infoDictionary];\n NSDictionary *RootDict = infoPlistDict[@\"Root\"];\n NSString *clientkey = RootDict[@\"clientKey\"];\n NSString *appId = RootDict[@\"appId\"];\n ICConfig *icConfig = [[ICConfig alloc] initWithClientKey:clientkey forAppId:appId];\n NSError *error;\n [IMIconnect startupWithConfig:icConfig error:&error];\n}\n",
"language": "objectivec"
}
]
}
[/block]
[block:api-header]
{
"type": "basic",
"title": "Setup APNs"
}
[/block]
This section will guide you through the configuration process for the Apple Push Notification service (APNs).
1. Go to the [Apple Developer Portal](https://developer.apple.com/) and login to your account.
2. Proceed to **Certificates, Identifiers & Profiles**.
3. Click Certificates -> All.
4. Click the **+** button to add a new certificate.
[block:image]
{
"images": [
{
"image": [
"https://files.readme.io/IiqHdvKSRuG5IOYwKFTD_4-0.png",
"4-0.png",
"982",
"216",
"#ededef",
""
],
"border": true
}
]
}
[/block]
5. Select the **Apple Push Notification service SSL (Sandbox & Production)** option.
[block:image]
{
"images": [
{
"image": [
"https://files.readme.io/bcc8908-NewPushCertificate.png",
"NewPushCertificate.png",
680,
254,
"#f1e7e6"
],
"border": true
}
]
}
[/block]
6. Select your App ID from the drop-down list and click Continue.
[block:image]
{
"images": [
{
"image": [
"https://files.readme.io/6Z1H8kZLTOGJTE0q8KPq_4-2.png",
"4-2.png",
"986",
"568",
"#9e5437",
""
]
}
]
}
[/block]
[block:api-header]
{
"type": "basic",
"title": "Create Certificate Signing Request (CSR)"
}
[/block]
1. Follow the on screen instructions to create a Certificate Signing Request.
2. Click **Continue**.
[block:image]
{
"images": [
{
"image": [
"https://files.readme.io/6cd03fa-CreateCSR.png",
"CreateCSR.png",
978,
721,
"#f0f1f1"
],
"border": true
}
]
}
[/block]
3. Click **Choose File** and select the certificate file that was created in the previous step.
[block:image]
{
"images": [
{
"image": [
"https://files.readme.io/LitSTR0nS26TJjS73v2T_4-6.png",
"4-6.png",
"982",
"558",
"#2db4e4",
""
],
"border": true
}
]
}
[/block]
4. Click **Generate**.
###Download, install & export the certificate
****
Once the APNs SSL certificate has been generated it must be downloaded, installed to your Keychain and exported as a .p12 file.
1. Click **Download** to download the certificate.
[block:image]
{
"images": [
{
"image": [
"https://files.readme.io/8ecc7fe-DownloadCert.png",
"DownloadCert.png",
954,
491,
"#eff1f1"
]
}
]
}
[/block]
2. Open the certificate to install it to your Keychain.
3. Within Keychain Access:
* Select the **login** keychain and the category as **My Certificates**.
* Locate and select the certificate.
* Right-click and select **Export** <your certificate>.
* Save the certificate as a *.p12* file.
[block:image]
{
"images": [
{
"image": [
"https://files.readme.io/YZTbXLnNQMGcrBkEQK8E_4-8.png",
"4-8.png",
"1155",
"469",
"#2e5a8b",
""
],
"border": true
}
]
}
[/block]
[block:callout]
{
"type": "info",
"body": "The exported .p12 file will be used later when configuring your app within IMIconnect."
}
[/block]
[block:api-header]
{
"title": "Firebase Cloud Messaging"
}
[/block]
If you wish to use Firebase Cloud Messaging (FCM) please refer to the official [FCM Guide](https://firebase.google.com/docs/cloud-messaging/ios/client) and add ``GoogleService-Info.plist`` in your project.
[block:api-header]
{
"type": "basic",
"title": "Add a Mobile Application"
}
[/block]
The section guides you through the process of configuring your app within the IMIconnect portal.
1. Login to your IMIconnect Portal using the URL provided to you when your tenant was created.
2. Click **Apps**.
3. Click **CONFIGURE APP** > **MOBILE / WEB**.
[block:image]
{
"images": [
{
"image": [
"https://files.readme.io/aa4fc41-addnewappw.png",
"addnewappw.png",
941,
408,
"#e7e2e2"
],
"border": true
}
]
}
[/block]
4. Enter a name for your application and click **NEXT**.
[block:image]
{
"images": [
{
"image": [
"https://files.readme.io/u5H8H4zgSEyhtxWSgfmO_sampleapp.png",
"sampleapp.png",
"507",
"175",
"#ef6392",
""
],
"sizing": "original"
}
]
}
[/block]
4. Select the Mobile platform and click **NEXT**.
[block:image]
{
"images": [
{
"image": [
"https://files.readme.io/h6F7QSfNQLicC2zd5rFi_selectmobile.png",
"selectmobile.png",
"578",
"229",
"#6d9cd8",
""
]
}
]
}
[/block]
5. Select the iOS platform and click **NEXT**.
6. The iOS platform specific features are displayed. Configure the options as per the table below:
[block:parameters]
{
"data": {
"h-1": "Settings",
"h-0": "Feature",
"0-0": "Real-time messaging",
"1-0": "Push messaging",
"2-0": "Two factor authentication",
"3-0": "Device attributes",
"0-1": "Configure the following:\n * Push messaging feature\n * RTM Transport and Security settings\n\n**Note**: The RTM Transport and Security settings are common for all app platforms. The settings you provide for one platform (e.g. iOS), also apply for all other platforms.\n\n**Transport protocols**\nTwo transport protocols are available for establishing a connection with IMIconnect, Web Socket and MQTT. If connection fails on the primary protocol, it will fall back to the secondary protocol. \n\n**Security settings**\nEnable secured ports to ensure RTM connections are established over a secured protocol for better security.\n\nEnable RTM payload encryption to encrypt the RTM payload in transit.",
"1-1": "If using APNs, select 'Apple Push Notification Service (APNs)' and configure the following:\n\n* Upload the APNs certificate generated in the previous section.\n* If the certificate is password protected, enter the password.\n* Select the Gateway (Production/Sandbox).\n\nSet to Production when sending push to your production ready app. To target your development app set to Sandbox.\n\nIf using FCM, select 'Firebase Cloud Messaging (FCM)' and configure the following:\n\n* Enter the FCM API Key as generated in the preceding section.",
"2-1": "Select the Sender ID through which the OTP (One Time Password) is sent.",
"3-1": "To monitor different attributes such as Time Zone, Device IP, RAM, Location and so on."
},
"cols": 2,
"rows": 2
}
[/block]
[block:image]
{
"images": [
{
"image": [
"https://files.readme.io/34273fe-Screenshot_2018-12-14_at_17.21.58.png",
"Screenshot 2018-12-14 at 17.21.58.png",
998,
246,
"#f9f7f6"
]
}
]
}
[/block]
7. Click **Save**.
8. Make a note of the **Client Key** that is displayed within the **Client Settings section. This key will be used while integrating the IMIconnect SDK within your application.
9. Click **Save**.
10. An overview screen appears with the newly created application profile and the corresponding App ID. Make a note of App Id for use during SDK integration.
[block:image]
{
"images": [
{
"image": [
"https://files.readme.io/d32faea-appidcreation.png",
"appidcreation.png",
866,
372,
"#e4e4e3"
]
}
]
}
[/block]
[block:api-header]
{
"type": "basic",
"title": "Code Integration"
}
[/block]
Follow the steps below to integrate the IMIconnect SDK within your application:
a. [Register a device](#section-c-register-a-device)
b. [Receive messages](#section-d-receive-messages)
c. [Connect to IMIconnect](#section-e-connect-to-imiconnect)
d. [Listen for connection status events](#section-f-listen-for-connection-status-events)
e. [Fetch Topics](#section-g-fetch-topics)
f. [Subscribe to a Topic](#section-h-subscribe-to-a-topic)
g. [Unsubscribe from a Topic](#section-i-unsubscribe-from-a-topic)
h. [Create a Thread](#section-j-create-a-thread)
i. [Publish a Message](#section-k-publish-a-message)
J. [Disconnect from IMIconnect](#section-l-disconnect-from-imiconnect)
###a. Register a device
***
The user's device must be registered with the IMIconnect platform before other features can be used. To register a device with the platform, create a ``ICDeviceProfile`` instance and invoke the IMIconnect.register method.
A device profile must always have a unique device id and user id, if you do not supply a user id then the platform will assign one for you. Typically you will want to supply your own user id value that corresponds to a user within your backend systems.
The following snippet shows how to create and register a device profile.
[block:code]
{
"codes": [
{
"code": "var deviceProfile = new ICDeviceProfile();\ndeviceProfile.setAppUserId(\"9999\");\ndeviceProfile.setDeviceId(\"PROVIDE UNIQUE DEVICE ID\");\nIMIconnectPlugin.register(\n function(result) {\n console.log(result);\n },\n function(error) {\n console.log(error);\n },\n deviceProfile\n);",
"language": "javascript",
"name": "Register a device"
}
]
}
[/block]
[block:callout]
{
"type": "info",
"body": "In a real world scenario, you will typically want to register with IMIconnect after a user has successfully logged into your app.",
"title": "Info:"
}
[/block]
[block:callout]
{
"type": "warning",
"body": "If your solution uses provisioned customer profiles (a.k.a master profiles), you will need to call ``IMIconnect.updateDeviceProfileParam`` to link the device profile to the customer profile. You may do this after successful device registration.\n\nSee [here](doc:createprofile) for information on provisioning customer profiles."
}
[/block]
###b. Receive messages
***
Incoming Push and In-App messages are received by registering a MessageListener implementation with IMIconnectPlugin.
The delegate also receives changes to the status of the In App messaging connection.
[block:code]
{
"codes": [
{
"code": "IMIconnectPlugin.messageListener(\n\n function(messages) {\n\n var text = \"\";\n for (var i = 0; i < messages.length; i++) {\n text += messages[i].getMessage() + \"<br>\";\n }\n\n console.log(text);\n\n });",
"language": "javascript",
"name": "Message Listner"
}
]
}
[/block]
###c. Connect to IMIconnect
***
To use In-App Messaging you must establish a connection with the IMIconnect platform by invoking the connect method.
Once the RT feature is enabled in the app asset created on IMIconnect and user registration is done, the App Developer can establish a connection between the app and IMIconnect platform by calling the **connect** method appropriately. This enables the messages sent from IMIconnect to be received on the app. When the application is running in the background, SDK is disconnected from IMIconnect. While in the disconnected state, incoming In-App messages are not received, however when the application comes to foreground again, SDK will establish a connection with IMIconnect platform and allow messages to be received.
[block:code]
{
"codes": [
{
"code": "IMIconnectPlugin.connect(\n function(result) {\n console.log(result);\n },\n function(error) {\n console.log(error);\n }\n);",
"language": "javascript",
"name": "IMIconnectPlugin"
}
]
}
[/block]
[block:callout]
{
"type": "info",
"title": "",
"body": "This method will return an error when In App Messaging is not enabled for the app or a device is not registered with the SDK."
}
[/block]
###d. Listen for connection status events
Events are raised by the SDK whenever the connection status has changed. To receive these events in your application; implement and register a ``connectionStatusChangedListener``.
[block:code]
{
"codes": [
{
"code": "IMIconnectPlugin.connectionStatusChangedListener(\n function(result) {\n console.log(result);\n },\n function(error) {\n console.log(error);\n });",
"language": "javascript",
"name": "connectionStatusChangedListener"
}
]
}
[/block]
###e. Fetch Topics
***
IMIconnect supports Topic based messaging, use the ``fetchTopics`` method to retrieve a list of topics configured for your application. You can subscribe users, or publish messages to those topics.
[block:callout]
{
"type": "info",
"title": "Info:",
"body": "Topics can be configured by an Admin in admin console of IMIconnect."
}
[/block]
[block:code]
{
"codes": [
{
"code": "IMIconnectPlugin.fetchTopics(function(topics) {\n var text = \"\";\n for (var i = 0; i < topics.length; i++) {\n text += topics[i].getName() + \"<br>\";\n }\n window.alert(text)\n\n}, function(error) {\n console.log(error);\n}, {\n \"offset\": 0\n});",
"language": "javascript",
"name": "fetchTopic"
}
]
}
[/block]
###f. Subscribe to a topic
***
Invoke the subscribe method to subscribe the current user to a topic.
[block:code]
{
"codes": [
{
"code": "IMIconnectPlugin.subscribeTopic(\n function(result) {\n console.log(result);\n },\n function(error) {\n console.log(error);\n },\n topicId);",
"language": "javascript",
"name": "subscribeTopic"
}
]
}
[/block]
###g. Unsubscribe from a topic
***
Invoke the unsubscribeTopic method to unsubscribe the current user from a topic.
[block:code]
{
"codes": [
{
"code": "IMIconnectPlugin.unsubscribeTopic(\n function(result) {\n console.log(result);\n },\n function(error) {\n console.log(error);\n },\n topicId);",
"language": "javascript",
"name": "unsubscribeTopic"
}
]
}
[/block]
Invoke the subscribe method to subscribe the current user to a topic.
###h. Create a thread
***
All In-App Messages within IMIconnect are grouped by threads. In order to publish messages, you must first create an ``ICThread`` object.
When responding to an incoming message, you can obtain the ``ICThread`` directly from the incoming message..
[block:code]
{
"codes": [
{
"code": "var thread = new ICThread();\nthread.setTitle(\"MyTitle\");\nthread.setCategory(\"MyCategory\");\nIMIconnectPlugin.createThread(function(thread) {\n window.alert(thread.getTitle());\n}, function(error) {\n console.log(error);\n}, thread);",
"language": "javascript",
"name": "Create a Thread"
}
]
}
[/block]
###i. Publish a message
***
Invoke publishMessage to publish a message to IMIconnect.
[block:code]
{
"codes": [
{
"code": "var message = new ICMessage();\nmessage.setMessage(\"Test Message\");\nmessage.setThread(thread);\nIMIconnectPlugin.publishMessage(function(icMessage) {\n console.log(JSON.stringify(icMessage.toJSON()));\n}, function(error) {\n console.log(error);\n}, message);",
"language": "javascript",
"name": "Publish Message"
}
]
}
[/block]
###j. Disconnect from IMIconnect
***
This method is used to disconnect from IMIconnect. Once disconnected, incoming In-App messages will not be received.
[block:code]
{
"codes": [
{
"code": "IMIconnectPlugin.disconnect(\n function(result) {\n console.log(result);\n },\n function(error) {\n console.log(error);\n }\n);",
"language": "javascript"
}
]
}
[/block]
[block:api-header]
{
"type": "basic",
"title": "Conclusion"
}
[/block]
Now you have completed your app integration with IMIconnect. Once you build your app and distribute, your app users can send and receive messages.
[block:api-header]
{
"title": "Rich Notification Support"
}
[/block]
[block:callout]
{
"type": "warning",
"title": "Note:",
"body": "This section is only required if you intend to use rich notifications."
}
[/block]
Rich notifications allow media, such as large images, to be displayed within the notification centre.
##Add dependancies
1. Drag-drop the ``IMIconnectNotificationServiceExtension.framework`` file into your XCode project.
2. Select **Create groups** and** Copy if needed** in the prompt that appears.
3. Click your project within the navigator and select your App Target. Click the **General** tab and add the ``IMIconnectNotificationServiceExtension.framework`` to the Embedded Binaries section.
4. Under the Capabilities tab, find and switch on "Keychain Sharing", and add a Keychain Group key (com.myappdomain.myappname). Use your application bundleIdentifier for Unique key.
5. Replicate the same for the extension target. Ensure that the Keychain Group key is the same for both - the app and the extension.
[block:image]
{
"images": [
{
"image": [
"https://files.readme.io/b9b0a0a-Keychain_Sharing.png",
"Keychain Sharing.png",
1852,
512,
"#fafafb"
],
"caption": "Keychain Sharing",
"border": true
}
]
}
[/block]
##Create an App Group
1. From the [Apple Developer Portal](https://developer.apple.com/), click **Certificates, Identifiers and Profiles**.
2. In the Identifiers section, select App Groups; click on the '**+**' button.
[block:image]
{
"images": [
{
"image": [
"https://files.readme.io/d14cb60-img1.jpg",
"img1.jpg",
553,
42,
"#e3e3e3"
],
"border": true,
"caption": "Add App Groups"
}
]
}
[/block]
3. Enter details in the fields - description of the group and unique identifier. Click on Continue.
[block:image]
{
"images": [
{
"image": [
"https://files.readme.io/86b5223-img2.jpg",
"img2.jpg",
553,
516,
"#eaeaec"
],
"caption": "Register an App Group"
}
]
}
[/block]
4. Click on **Register** and **Done**.
[block:image]
{
"images": [
{
"image": [
"https://files.readme.io/21aa93e-img3.jpg",
"img3.jpg",
553,
523,
"#e7e7e8"
],
"border": true,
"caption": "Confirm App Group"
}
]
}
[/block]
##Create a Notification Service Extension
1. Within XCode, click your **project** within the **Project Navigator**.
2. Within the **Targets** section, click the '**+**' button.
[block:image]
{
"images": [
{
"image": [
"https://files.readme.io/da0db87-img5.jpg",
"img5.jpg",
232,
537,
"#dcdade"
],
"border": true,
"caption": "Add Target"
}
]
}
[/block]
3. Within the **Application Extension** section, select **Notification Service Extension** and click **Next**.
[block:image]
{
"images": [
{
"image": [
"https://files.readme.io/adbb6b7-img6.jpg",
"img6.jpg",
553,
398,
"#ecebef"
],
"border": true,
"caption": "Notification Service Extension"
}
]
}
[/block]
4. Enter a name and click **Finish**.
5. Click **Activate**.
[block:image]
{
"images": [
{
"image": [
"https://files.readme.io/386332e-img7.jpg",
"img7.jpg",
553,
308,
"#efeaf0"
],
"caption": "Activate Push Notification Service Extension",
"border": true
}
]
}
[/block]
6. You should now see a new folder in your project hierarchy containing three new files -
**NotificationService.h**, **NotificationService.m**, and **Info.plist**.
[block:image]
{
"images": [
{
"image": [
"https://files.readme.io/91d2f23-img8.jpg",
"img8.jpg",
347,
577,
"#f2f0ef"
],
"border": true,
"caption": "Folder Hierarchy"
}
]
}
[/block]
7. Click your **project** within the **Project Navigator**.
8. Select your notification service extension target.
9. Click the **Capabilities** tab.
10. Switch on the App Group and click on the app group that you created earlier.
Repeat steps 9 and 10 for your main application target.
[block:image]
{
"images": [
{
"image": [
"https://files.readme.io/c6a896a-AppGroup.png",
"AppGroup.png",
820,
158,
"#fafafb"
],
"caption": "Select Notification Service Extension Target"
}
]
}
[/block]
11. Select the **Build Phases** tab for the Notification Service Extension and in the **Link Binary with Libraries** section, add **IMIconnectNotificationServiceExtension.framework**.
[block:image]
{
"images": [
{
"image": [
"https://files.readme.io/b5e6427-Link_Binary.png",
"Link Binary.png",
552,
162,
"#f9f9f9"
],
"border": true
}
]
}
[/block]
12. Remove the default code that was generated for **NotificationService**, leave only the class definition.
13. Change your **NotificationService** to inherit from **ICNotificationService**.
[block:code]
{
"codes": [
{
"code": "//Within NotificationService.h\n\n#import <UserNotifications/UserNotifications.h>\n\n#import <IMIconnectNotificationServiceExtension/IMIconnectNotificationServiceExtension.h>\n\n@interface NotificationService : ICNotificationService\n\n@end\n\n\n//Within NotifcationService.m\n#import \"NotificationService.h\"\n \n@interface NotificationService ()\n\n\n@end\n\n@implementation NotificationService\n\n\n\n@end\n",
"language": "objectivec"
},
{
"code": "import UserNotifications\n\nclass NotificationService: ICNotificationService\n{\n \n}",
"language": "swift"
}
]
}
[/block]
[block:image]
{
"images": [
{
"image": [],
"border": true,
"caption": "Add File to Notification Service Extension target"
}
]
}
[/block]
14. If you are not using **IMIconnectConfiguration.plist** open **NotificationService.m** and override the getter to return your app group identifier.
[block:code]
{
"codes": [
{
"code": "#import \"NotificationService.h\"\n\n@implementation NotificationService\n- (NSString *)groupIdentifier\n{\nreturn @\"<Your app group identifier>\";\n}\n\n@end",
"language": "objectivec",
"name": "Sample Code"
}
]
}
[/block]
Updated over 2 years ago